OverTheWire Krypton Levels 0 to 5 Walkthrough
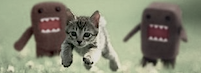
Walkthrough on solving the Krypton series from the wargame site, OverTheWire
I’ve avoided crypto challenges in CTF events I’ve participated in the past because I felt like it was one of my weaker areas of knowledge, but I decided to change. So I’ve attempted the Krypton challenge series by overthewire.org as a way to begin strengthening this area of knowledge.
Level 0
When we first access the Krypton page for level 0 we are greeted with the following message.
Level Info
Welcome to Krypton! The first level is easy. The following string encodes the password using Base64:
S1JZUFRPTklTR1JFQVQ=
Use this password to log in to krypton.labs.overthewire.org with username krypton1 using SSH. You can the files for other levels in /krypton/
So we have to decode the base64 string “S1JZUFRPTklTR1JFQVQ=” into the plaintext password to find the password for krypton1. There are many different ways you can solve this challenge. I decided to write a quick python script which would take the base64 string and decode it and print the plaintext password to the screen.
#!/usr/bin/python
import base64
keyin = "S1JZUFRPTklTR1JFQVQ="
keyout = base64.b64decode(keyin)
print(keyout)
Level 1
Once logged into the level1 account on krypton.labs.overthewire.org, I went to the krypton1 directory and saw that there were 2 files.
krypton1@melinda:/krypton/krypton1$ ls -lh
total 2.0K
-rw-r----- 1 krypton1 krypton1 882 Jun 6 2013 README
-rw-r----- 1 krypton1 krypton1 26 Jun 6 2013 krypton2
krypton1@melinda:/krypton/krypton1$ cat README
Welcome to Krypton!
This game is intended to give hands on experience with cryptography
and cryptanalysis. The levels progress from classic ciphers, to modern,
easy to harder.
Although there are excellent public tools, like cryptool,to perform
the simple analysis, we strongly encourage you to try and do these
without them for now. We will use them in later excercises.
** Please try these levels without cryptool first **
The first level is easy. The password for level 2 is in the file
'krypton2'. It is 'encrypted' using a simple rotation called ROT13.
It is also in non-standard ciphertext format. When using alpha characters for
cipher text it is normal to group the letters into 5 letter clusters,
regardless of word boundaries. This helps obfuscate any patterns.
This file has kept the plain text word boundaries and carried them to
the cipher text.
Enjoy!
krypton1@melinda:/krypton/krypton1$ cat krypton2
YRIRY GJB CNFFJBEQ EBGGRA
So the README file explains this challenge and the krypton2 file contains the password for the next level which is encrypted using a ROT13 cipher. In python, we can use string methods to decode or encode a string. And if we look at the support encoding codecs for these methods, we can see that ROT13 is one of them.
https://docs.python.org/2/library/stdtypes.html https://docs.python.org/2/library/codecs.html#codec-base-classes
Which means all we have to do is solve this problem is the following string.
"YRIRY GJB CNFFJBEQ EBGGRA".decode(encoding="ROT13")
u'LEVEL TWO PASSWORD ******'
Level 2
Once logged into the level1 account on krypton.labs.overthewire.org, I went to the krypton2 directory and saw that there were 4 files.
krypton2@melinda:/krypton/krypton2$ ls -lh
total 13K
-rw-r----- 1 krypton2 krypton2 1.1K Jun 6 2013 README
-rwsr-x--- 1 krypton3 krypton2 8.7K Jun 6 2013 encrypt
-rw-r----- 1 krypton3 krypton3 27 Jun 6 2013 keyfile.dat
-rw-r----- 1 krypton2 krypton2 13 Jun 6 2013 krypton3
krypton2@melinda:/krypton/krypton2$ cat README
Krypton 2
ROT13 is a simple substitution cipher.
Substitution ciphers are a simple replacement algorithm. In this example
of a substitution cipher, we will explore a 'monoalphebetic' cipher.
Monoalphebetic means, literally, "one alphabet" and you will see why.
This level contains an old form of cipher called a 'Caesar Cipher'.
A Caesar cipher shifts the alphabet by a set number. For example:
plain: a b c d e f g h i j k ...
cipher: G H I J K L M N O P Q ...
In this example, the letter 'a' in plaintext is replaced by a 'G' in the
ciphertext so, for example, the plaintext 'bad' becomes 'HGJ' in ciphertext.
The password for level 3 is in the file krypton3. It is in 5 letter
group ciphertext. It is encrypted with a Caesar Cipher. Without any
further information, this cipher text may be difficult to break. You do
not have direct access to the key, however you do have access to a program
that will encrypt anything you wish to give it using the key.
If you think logically, this is completely easy.
One shot can solve it!
Have fun.
So the password in the krypton3 file is encrypted using a Caesar Cipher. And as you can see the from the example, in a Caesar Cipher the Alphabet is shifted once given by a certain number. If we can work out what number was used to shift the Alphabet we can decrypt the message. We know that the encrypt ELF binary, was used to encrypt the message.
There are several methods I could use to work out the shift value:
- Reverse Engineering – Opening the application in IDA or GDB and find the shift process and observe the shift occurring.
- I can put the entire Alphabet in sequence and then run it through the encrypt binary to try and produce a key for the message.
- Or brute-force the key for the cipher.
I decided to go with the brute-force approach. Using python I created the below script which can be used to crack the decryption key and reveal the plaintext messaged.
#!/usr/bin/python
import sys
ctext = "OMQEMDUEQMEK"
alphabet = list("ABCDEFGHIJKLMNOPQRSTUVWXYZ")
plaintext = ""
shift = 1
while shift <= 26:
for c in ctext:
if c in alphabet:
plaintext += alphabet[(alphabet.index(c)+shift)%(len(alphabet))]
print("Shift used: " + str(shift))
print("Ciphertext: " + ctext)
print("Plaintext: " + plaintext)
shift = shift + 1
plaintext = ""
krypton2@melinda:/tmp/kryptontest$ ./caesar.py
-- omitted output --
Shift used: 10
Ciphertext: OMQEMDUEQMEK
Plaintext: YWAOWNEOAWOU
Shift used: 11
Ciphertext: OMQEMDUEQMEK
Plaintext: ZXBPXOFPBXPV
Shift used: 12
Ciphertext: OMQEMDUEQMEK
Plaintext: AYCQYPGQCYQW
Shift used: 13
Ciphertext: OMQEMDUEQMEK
Plaintext: BZDRZQHRDZRX
Shift used: 14
Ciphertext: OMQEMDUEQMEK
Plaintext: ************
Shift used: 15
Ciphertext: OMQEMDUEQMEK
Plaintext: DBFTBSJTFBTZ
-- omitted output --
The brute-force for Caesar Ciphers is a viable choice because the maximum amount of attempts needed to brute-force when using the English Alphabet is 26. Which is why I had a While loop in my brute-forcing script to continue trying until shift is equal to 26. You can also see from the output from the script, that the alphabet was had been shifted 14 places.
Level 3
krypton3@melinda:/krypton/krypton3$ ls -lh
total 10K
-rw-r----- 1 krypton3 krypton3 56 Jun 6 2013 HINT1
-rw-r----- 1 krypton3 krypton3 37 Jun 6 2013 HINT2
-rw-r----- 1 krypton3 krypton3 785 Jun 6 2013 README
-rw-r----- 1 krypton3 krypton3 1.6K Jun 6 2013 found1
-rw-r----- 1 krypton3 krypton3 2.1K Jun 6 2013 found2
-rw-r----- 1 krypton3 krypton3 560 Jun 6 2013 found3
-rw-r----- 1 krypton3 krypton3 42 Jun 6 2013 krypton4
krypton3@melinda:/krypton/krypton3$ cat README
Well done. You've moved past an easy substitution cipher.
Hopefully you just encrypted the alphabet a plaintext
to fully expose the key in one swoop.
The main weakness of a simple substitution cipher is
repeated use of a simple key. In the previous exercise
you were able to introduce arbitrary plaintext to expose
the key. In this example, the cipher mechanism is not
available to you, the attacker.
However, you have been lucky. You have intercepted more
than one message. The password to the next level is found
in the file 'krypton4'. You have also found 3 other files.
(found1, found2, found3)
You know the following important details:
- The message plaintexts are in English (*** very important)
- They were produced from the same key (*** even better!)
Enjoy.
Before I looked at the found files, I decided to have a look at the hints.
krypton3@melinda:/krypton/krypton3$ cat HINT1
Some letters are more prevalent in English than others.
krypton3@melinda:/krypton/krypton3$ cat HINT2
"Frequency Analysis" is your friend.
So it looks like, we’ll have a to perform “Frequency Analysis” on the the found files. What Frequency Analysis is, some letters appear more frequency in English words the others. From my prior studies and research, I know ‘e’ is one of the most frequently used letters in the English language.
I submitted the found1 ciphertext to this website, to get a better idea of the frequency of letters. http://www.richkni.co.uk/php/crypta/freq.php
The report tells me the following information:
- The single most frequent letter is “s”.
- The most frequent 3 letter squence is “jds”.
And with the aid of this hints and tips document from “The Black Chamber”, http://www.simonsingh.net/The_Black_Chamber/hintsandtips.html
ENGLISH
Order Of Frequency Of Single Letters
E T A O I N S H R D L U
Order Of Frequency Of Digraphs
th er on an re he in ed nd ha at en es of or nt ea ti to it st io le is ou ar as de rt ve
Order Of Frequency Of Trigraphs
the and tha ent ion tio for nde has nce edt tis oft sth men
Order Of Frequency Of Most Common Doubles
ss ee tt ff ll mm oo
Order Of Frequency Of Initial Letters
T O A W B C D S F M R H I Y E G L N P U J K
Order Of Frequency Of Final Letters
E S T D N R Y F L O G H A K M P U W
One-Letter Words
a, I
Most Frequent Two-Letter Words
of, to, in, it, is, be, as, at, so, we, he, by, or, on, do, if, me, my, up, an, go, no, us, am
Most Frequent Three-Letter Words
the, and, for, are, but, not, you, all, any, can, had, her, was, one, our, out, day, get, has, him, his, how, man, new, now, old, see, two, way, who, boy, did, its, let, put, say, she, too, use
Most Frequent Four-Letter Words
that, with, have, this, will, your, from, they, know, want, been, good, much, some, time
I can assume that most likely “s” in the ciphertext is actually “e” in plaintext and “jds” in the ciphertext is actually the word “the”. And if “s” is actually the “e” in plaintext, it would support the idea of the sequence “jds” being the word “the”. And continuing on this assumption I worked out the following information:
- In the ciphertext “s” equals “e” in plaintext.
- The letter sequence in the ciphertext “jds” is likely “the” in plaintext.
- The letter “j” in the cipher is likely letter “t” in plaintext.
- The letter “d” in the cipher is likely letter “h” in plaintext.
- The sequence “su” in the cipher is likely “es” in plaintext.
- The sequence “jc” in the cipher is likely “ti” in plaintext.
- The letter “q” in the cipher is likely “a” in plaintext.
- The sequence “qn” in the cipher is likely “ar” in plaintext.
- The sequence “qg” in the cipher is likely “an” in plaintext.
- The sequence “qgw” in the cipher is likely “and” in plaintext if “qg” is “an”.
- The sequence “cbg” in the cipher is likely “ion” in plaintext.
- The letter “x” in the cipher is likely to be “f” in plaintext because of “bx” is likely to be “of”.
- The sequence “cge” in the cipher is likely “ing” in plaintext.
- The sequence “vv” in the cipher is likely “ll” in plaintext because with all the information above it can only be “l” or “m”.
- The sequence “zcydsn” in the ciphertext is likely to be “cipher”. *
- The sequence “zqsuqn” in the ciphertext is likely to be “caesar”.
- The letter “l” in the ciphertext is liekly to be “y”. Because in the currently worked out letters of the alphabet, the ciphertext “cgznl yjben qydl” is “INCRl PTOGR APHl” in plaintext and if you change the “l” to a “y” it becomes “IN CRYPTOGRAPHY”.
- The letter “f” in the ciphertext is likely to be the letter “k” in the plaintext. **
- The letter “k” in the ciphertext is likely to be the letter “w” in the plaintext. **
- The letter “t” in the ciphertext is likely to be the letter “m” in the plaintext. **
- The letter “h” in the ciphertext is likely to be the letter “q” in the plaintext. **
- The letter “m” in the ciphertext is likely to be the letter “u” in the plaintext. **
- The letter “a” in the ciphertext is likely to be the letter “b” in the plaintext. **
- The letter “o” in the ciphertext is likely to be the letter “x” in the plaintext. **
NOTE: * Using words with friends cheating websites I was able to perform character (?/*) substitution. And out of the all the 5 letter words generated, I thought “cipher” would fit the context of this message the best. ** These letters were worked out by reading the first line of the ciphertext which had been partially worked out already, and testing what letters would be fit.
IN CRYPTOGRAPHY A CAESAR CIPHER ALSO KNOWN AS A CAESAR'S CIPHER, THE SHIFT CIPHER, CAESAR'S CODE OR CAESAR SHIFT IS ONE OF THE SIMPLEST AND MOST WIDELY KNOWN ENCRYPTION TECHNIQUES IT IS A TYPE OF SUBSTITUTION CIPHER IN WHICH EACH LETTER IN THE PLAINTEXT IS REPLACED BY A LETTER SO tEF IoEDN mtaER OFPOS ITION SDOkN THEAL PHAaE TFORE oAtPL EkITH ASHIF TOFAk OmLDa EREPL ACEDa YDakO mLDaE COtEE ANDSO ONTHE tETHO DISNA tEDAF TERrm LImSC AESAR kHOmS EDITT OCOtt mNICA TEkIT HHISG ENERA LSTHE ENCRY PTION STEPP ERFOR tEDaY ACAES ARCIP HERIS OFTEN INCOR PORAT EDASP ARTOF tOREC OtPLE oSCHE tESSm CHAST HEiIG ENREC IPHER ANDST ILLHA StODE RNAPP LICAT IONIN THERO TSYST EtASk ITHAL LSING LEALP HAaET SmaST ITmTI ONCIP HERST HECAE SARCI PHERI SEASI LYaRO fENAN DINPR ACTIC EOFFE RSESS ENTIA LLYNO COttm NICAT IONSE CmRIT YSHAf ESPEA REPRO DmCED tOSTO FHISf NOkNk ORfaE TkEEN ANDHI SEARL YPLAY SkERE tAINL YCOtE DIESA NDHIS TORIE SGENR ESHER AISED TOTHE PEAfO FSOPH ISTIC ATION ANDAR TISTR YaYTH EENDO FTHES IoTEE NTHCE NTmRY NEoTH EkROT EtAIN LYTRA GEDIE SmNTI LAaOm TINCL mDING HAtLE TfING LEARA NDtAC aETHC ONSID EREDS OtEOF THEFI NESTE oAtPL ESINT HEENG LISHL ANGmA GEINH ISLAS TPHAS EHEkR OTETR AGICO tEDIE SALSO fNOkN ASROt ANCES ANDCO LLAaO RATED kITHO THERP LAYkR IGHTS tANYO FHISP LAYSk EREPm aLISH EDINE DITIO NSOFi ARYIN GhmAL ITYAN DACCm RACYD mRING HISLI FETIt EANDI NTkOO FHISF ORtER THEAT RICAL COLLE AGmES PmaLI SHEDT HEFIR STFOL IOACO LLECT EDEDI TIONO FHISD RAtAT ICkOR fSTHA TINCL mDEDA LLamT TkOOF THEPL AYSNO kRECO GNISE DASSH AfESP EARES
To get the final letters or so, I had started reading the message and replacing the letters which would best fit. After awhile I realised this was the word for word from the Wikapedia page for the Caesar Cipher. http://en.wikipedia.org/wiki/Caesar_cipher
IN CRYPTOGRAPHY A CAESAR CIPHER ALSO KNOWN AS A CAESAR'S CIPHER, THE SHIFT CIPHER CAESARS CODE OR CAESAR SHIFT IS ONE OF THE SIMPLEST AND MOST WIDELY KNOWN ENCRYPTION TECHNIQUES IT IS A TYPE OF SUBSTITUTION CIPHER IN WHICH EACH LETTER IN THE PLAINTEXT IS REPLACED BY A LETTER SOME FIXED NUMBER OF POSITIONS DOWN THE ALPHABET. FOR EXAMPLE WITH A SHIFT OF A WOULD BE REPLACED BY D B WOULD BE COME E AND SO ON. THE METHOD IS NAMED AFTER JULIUS CAESAR WHO USED IT TO COMMUNICATE WITH HIS GENERALS THE ENCRYPTION STEP PERFORMED BY A CAESAR CIPHER IS OFTEN INCORPORATED AS PART OF MORE COMPLEX SCHEMES SUCH AS THE VIGENRE CIPHER AND STILL HAS MODERN APPLICATION IN THE ROT SYSTEM AS WITH ALL SINGLE ALPHABET SUBSTITUTION CIPHERS THE CAESAR CIPHER IS EASILY BROKEN AND IN PRACTICE OFFERS ESSENTIALLY NO COMMUNICATION SECURITY.
SHAKESPEARE PRODUCED MOST OF HIS KNOWN WORK BETWEEN AND HIS EARLY PLAYS WERE MAINLY COMEDIES AND HISTORIES GENRES HE RAISED TO THE PEAK OF SOPHISTICATION AND ARTISTRY BY THE END OF THE SIXTEENTH CENTURY NEXT HE WROTE MAINLY TRAGEDIES UNTIL ABOUT INCLUDING HAMLET KING LEAR AND MACBETH CONSIDERED SOME OF THE FINEST EXAMPLES IN THE ENGLISH LANGUAGE IN HIS LAST PHASE HE WROTE TRAGICOMEDIES ALSO KNOWN AS ROMANCES AND COLLABORATED WITH OTHER PLAYWRIGHTS MANY OF HIS PLAYS WERE PUBLISHED IN EDITIONS OF VARYING QUALITY AND ACCURACY DURING HIS LIFETIME AND IN TWO OF HIS FORMER THEATRICAL COLLEAGUES PUBLISHED THE FIRST FOLIO A COLLECTED EDITION OF HIS DRAMATIC WORKS THAT INCLUDED ALL BUT TWO OF THE PLAYS NOW RECOGNISED AS SHAKESPEARES.
Continuing on I realised that there was a second part to the message, it was from the Shakespeare Wikipedia page. http://en.wikipedia.org/wiki/William_Shakespeare
We don’t actually need to decrypt the other 2 messages because they all were generated using the same key. Which means all we need to do is take the ciphertext in the krypton4 file and put it through the decryption and we’ll get the plaintext message.
KSVVW BGSJD SVSIS VXBMN YQUUK BNWCU ANMJS
WELL DONE THE LEVEL FOUR PASSWORD IS *****
For those wondering, I worked out the alphabet key to be the following.
Alphabet: a b c d e f g h i j k l m
Encryption: b o i h g k n q v i w y u
Alphabet: n o p q r s t u v w x y z
Encryption: r x z a j e m s l d f p c
Level 4
krypton4@melinda:/krypton/krypton4$ ls -lh
total 9.0K
-rw-r----- 1 krypton4 krypton4 287 Jun 6 2013 HINT
-rw-r----- 1 krypton4 krypton4 1.4K Jun 6 2013 README
-rw-r----- 1 krypton4 krypton4 1.7K Jun 6 2013 found1
-rw-r----- 1 krypton4 krypton4 2.9K Jun 6 2013 found2
-rw-r----- 1 krypton4 krypton4 10 Jun 6 2013 krypton5
krypton4@melinda:/krypton/krypton4$ cat README
Good job!
You more than likely used frequency analysis and some common sense
to solve that one.
So far we have worked with simple substitution ciphers. They have
also been 'monoalphabetic', meaning using a fixed key, and
giving a one to one mapping of plaintext (P) to ciphertext (C).
Another type of substitution cipher is referred to as 'polyalphabetic',
where one character of P may map to many, or all, possible ciphertext
characters.
An example of a polyalphabetic cipher is called a Vigenère Cipher. It works
like this:
If we use the key(K) 'GOLD', and P = PROCEED MEETING AS AGREED, then "add"
P to K, we get C. When adding, if we exceed 25, then we roll to 0 (modulo 26).
P P R O C E E D M E E T I N G A S A G R E E D
K G O L D G O L D G O L D G O L D G O L D G O
becomes:
P 15 17 14 2 4 4 3 12 4 4 19 8 13 6 0 18 0 6 17 4 4 3
K 6 14 11 3 6 14 11 3 6 14 11 3 6 14 11 3 6 14 11 3 6 14
C 21 5 25 5 10 18 14 15 10 18 4 11 19 20 11 21 6 20 2 8 10 17
So, we get a ciphertext of:
VFZFK SOPKS ELTUL VGUCH KR
This level is a Vigenère Cipher. You have intercepted two longer, english
language messages. You also have a key piece of information. You know the
key length!
For this exercise, the key length is 6. The password to level five is in the usual
place, encrypted with the 6 letter key.
Have fun!
krypton4@melinda:/krypton/krypton4$ cat HINT
Frequency analysis will still work, but you need to analyse it
by "keylength". Analysis of cipher text at position 1, 6, 12, etc
should reveal the 1st letter of the key, in this case. Treat this as
6 different mono-alphabetic ciphers...
Persistence and some good guesses are the key!
So for this challenge a Vigenere Cipher was used to encrypt plaintext and I’ll have to find out the key used to encrypt the plaintext. To being with I look at the found1 file. So we know the key length is 6 characters. The tells us Frequency analysis will still work but we’ll have to analysis via keylength, the following link explains why a vigenere cipher is stronger then a substitution cipher. [http://www.simonsingh.net/The_Black_Chamber/vigenere_strength.html)(http://www.simonsingh.net/The_Black_Chamber/vigenere_strength.html)
krypton4@melinda:/krypton/krypton4$ cat found1
YYICS JIZIB AGYYX RIEWV IXAFN JOOVQ QVHDL CRKLB SSLYX RIQYI IOXQT WXRIC RVVKP BHZXI YLYZP DLCDI IKGFJ UXRIP TFQGL CWVXR IEZRV NMYSF JDLCL RXOWJ NMINX FNJSP JGHVV ERJTT OOHRM VMBWN JTXKG JJJXY TSYKL OQZFT OSRFN JKBIY YSSHE LIKLO RFJGS VMRJC CYTCS VHDLC LRXOJ MWFYB JPNVR NWUMZ GRVMF UPOEB XKSDL CBZGU IBBZX MLMKK LOACX KECOC IUSBS RMPXR IPJZW XSPTR HKRQB VVOHR MVKEE PIZEX SDYYI QERJJ RYSLJ VZOVU NJLOW RTXSD LYYNE ILMBK LORYW VAOXM KZRNL CWZRA YGWVH DLCLZ VVXFF KASPJ GVIKW WWVTV MCIKL OQYSW SBAFJ EWRII SFACC MZRVO MLYYI MSSSK VISDY YIGML PZICW FJNMV PDNEH ISSFE HWEIJ PSEEJ QYIBW JFMIC TCWYE ZWLTK WKMBY YICGY WVGBS UKFVG IKJRR DSBJJ XBSWM VVYLR MRXSW BNWJO VCSKW KMBYY IQYYW UMKRM KKLOK YYVWX SMSVL KWCAV VNIQY ISIIB MVVLI DTIIC SGSRX EVYQC CDLMZ XLDWF JNSEP BRROO WJFMI CSDDF YKWQM VLKWM KKLOV CXKFE XRFBI MEPJW SBWFJ ZWGMA PVHKR BKZIB GCFEH WEWSF XKPJT NCYYR TUICX PTPLO VIJVT DSRMV AOWRB YIBIR MVWER QJKWK RBDFY MELSF XPEGQ KSPML IYIBX FJPXR ELPVH RMKFE HLEBJ YMWKM TUFII YSUXE VLJUX YAYWU XRIUJ JXGEJ PZRQS TJIJS IJIJS PWMKK KBEQX USDXC IYIBI YSUXR IPJNM DLBFZ WSIQF EHLYR YVVMY NXUSB SRMPW DMJQN SBIRM VTBIR YPWSP IIIIC WQMVL KHNZK SXMLY YIZEJ FTILY RSFAD SFJIW EVNWZ WOWFJ WSERB NKAKW LTCSX KCWXV OILGL XZYPJ NLSXC YYIBM ZGFRK VMZEH DSRTJ ROGIM RHKPQ TCSCX GYJKB ICSTS VSPFE HGEQF JARMR JRWNS PTKLI WBWVW CXFJV QOVYQ UGSXW BRWCS MSCIP XDFIF OLGSU ECXFJ PENZY STINX FJXVY YLISI MEKJI SEKFJ IEXHF NCPSI PKFVD LCWVA OVCSF JKVKX ESBLM ZJICM LYYMC GMZEX BCMKK LOACX KEXHR MVKBS SSUAK WSSKM VPCIZ RDLCF WXOVL TFRDL CXLRC LMSVL YXGSK LOMPK RGOWD TIXRI PJNIB ILTKV OIQYF SPJCW KLOQQ MRHOW MYYED FCKFV ORGLY XNSPT KLIEL IKSDS YSUXR IJNFR GIPJK MBIBF EHVEW IFAXY NTEXR IEWRW CELIW IVPYX CIOTU NKLDL CBFSN QYSRR NXFJJ GKVCH ISGOC JGMXK UFKGR
Because of the Vigenere Cipher is a polyalphabetic cipher making it resistant to frequency analysis. The following link, http://www.simonsingh.net/The_Black_Chamber/crackingprinciple.html, explains how to the cracking principle for attacking the Vigenere cipher.
Using the following website, http://smurfoncrack.com/pygenere/pygenere.php, and entered the found1 cipher, it was able to produce readable plaintext and also reported back that key was suspected to be: “FREKEY”
krypton4@melinda:/krypton/krypton4$ cat krypton5
HCIKV RJOX
Since we have the key and the ciphertext, we can work out the plaintext.
H C I K V R J O X
F R E K E Y F R E
* * * * * * * * *
Level 5
krypton5@melinda:/krypton/krypton5$ ls -lh
total 9.0K
-rw-r----- 1 krypton5 krypton5 151 Jun 12 2013 README
-rw-r----- 1 krypton5 krypton5 1.8K Jun 12 2013 found1
-rw-r----- 1 krypton5 krypton5 1.9K Jun 12 2013 found2
-rw-r----- 1 krypton5 krypton5 2.1K Jun 12 2013 found3
-rw-r----- 1 krypton5 krypton5 7 Jun 12 2013 krypton6
krypton5@melinda:/krypton/krypton5$ cat README
Frequency analysis can break a known key length as well. Lets try one
last polyalphabetic cipher, but this time the key length is unknown.
Enjoy.
Using the same website as before, http://smurfoncrack.com/pygenere/index.php, I can specify the length of the codeword to try but since I don’t know the length of the key used this time, we can specific to try a key length range. I decided to leave it at the default size of attempting to try keys with a length of 5 – 9. It returned the found1 ciphertext to readable plaintext and said the original codeword was most likely “keylength”. Then using the deciphering tool from this website, http://www.simonsingh.net/The_Black_Chamber/vigenere_square_tool.html, I can work out the plaintext.
B E L O S Z
K E Y L E N
* * * * * *
Level 6
Currently working on a solution for this level.